Debugging Tips for Frontend Developers (React + Next.js)
By Muhammad Sharjeel | Published on Sat Apr 26 2025
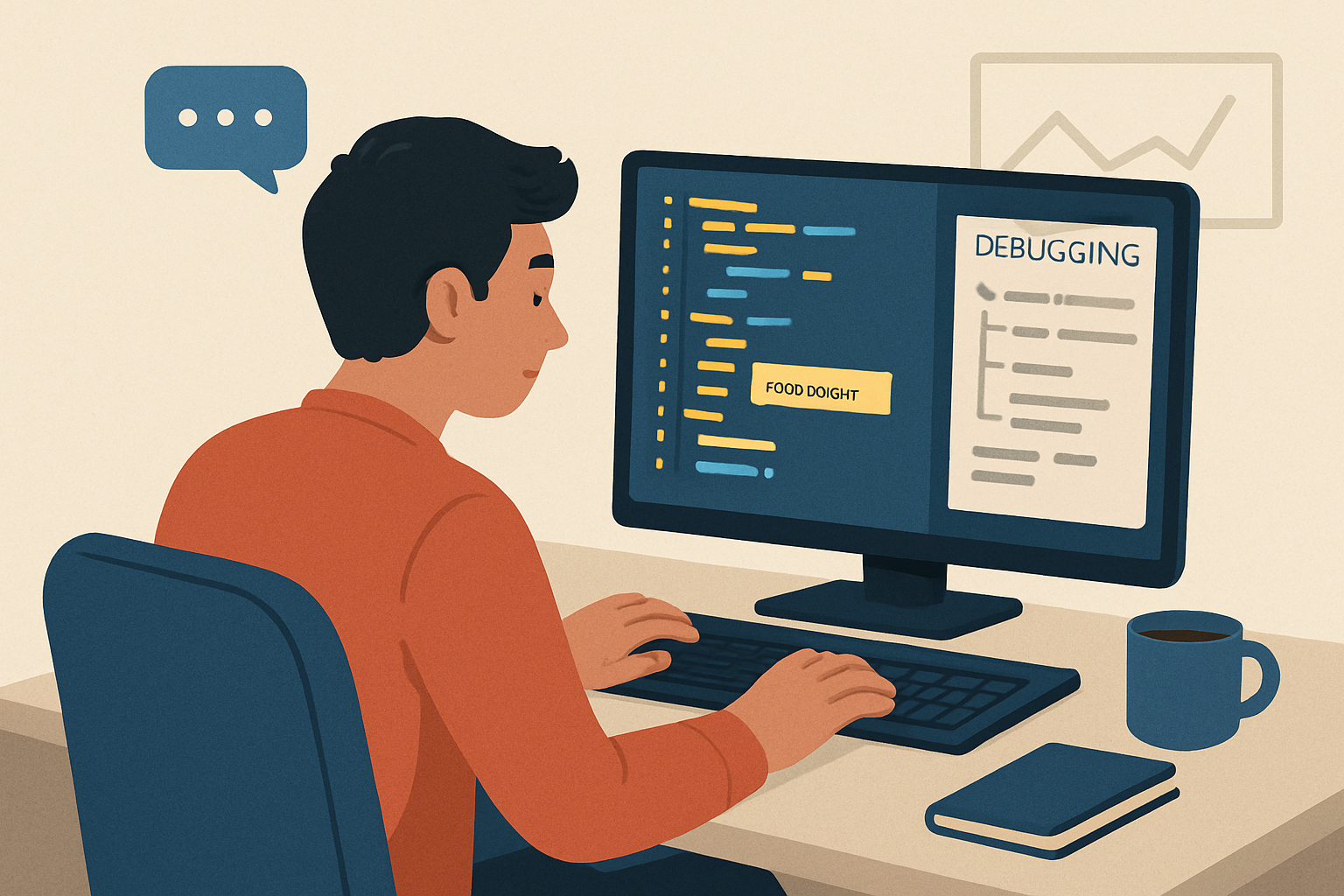
Why Debugging Mastery Matters
Debugging isn't just fixing what's broken — it's about understanding the full lifecycle of your application. The earlier you learn this mindset, the faster you’ll grow as a developer.
Debugging with Logs
Logging is your first and simplest line of defense. Use console.log
, console.error
, and console.warn
effectively to track what your code is doing at every step.
Example: Logging API Request/Response
async function fetchUserData() {
console.log("Starting API call...");
try {
const response = await fetch('/api/user');
const data = await response.json();
console.log("API call success:", data);
return data;
} catch (error) {
console.error("API call failed:", error);
}
}
Notice how we log before starting the call, and separately log the successful and error paths.
Using the debugger
Keyword
When logging isn’t enough, the debugger
keyword lets you pause code execution and inspect live values and the call stack in DevTools.
Example: Freezing the Code with Debugger
function calculateTotal(price, tax) {
const total = price + tax;
debugger; // Execution will pause here
return total;
}
const finalAmount = calculateTotal(100, 20);
console.log("Final Amount:", finalAmount);
When you reach debugger
, the browser will pause execution. You can inspect variables like price
, tax
, and total
right inside DevTools.
Debugging APIs and Network Issues
React and Next.js apps are API-heavy. Knowing how to debug API calls is crucial.
- Always log the request payload and API response.
- Check the Network tab in DevTools to inspect headers, status codes, and timings.
- Look for common mistakes: incorrect URLs, missing auth headers, wrong HTTP methods, or parsing errors.
Example: API Call Debugging
async function loginUser(credentials) {
console.log("Sending login request with credentials:", credentials);
try {
const res = await fetch('/api/login', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(credentials),
});
const result = await res.json();
console.log("Login response:", result);
} catch (error) {
console.error("Login API failed:", error);
}
}
Checking API Response Time
Use the DevTools Network tab to analyze:
- DNS Lookup
- Connection time
- Server processing time
- Download time
Identify if slowness is happening on your end (frontend) or backend (server).
Special Tips for React and Next.js
In React:
- Use
React Developer Tools
extension to inspect props and state visually. - Log inside
useEffect
to trace when effects re-run.
In Next.js:
- Use
console.log
insidegetServerSideProps
andAPI Routes
. - Remember that server-side logs appear in the Node.js terminal (not browser).
Real-World Debugging Scenario
Bug: Button Click Not Working
function MyButton() {
const handleClick = () => {
console.log("Button clicked!");
// Suppose something is supposed to happen here
};
return (
<button onClick={handleClick}>Click Me</button>
);
}
If clicking doesn't log anything:
- Check if the event handler is wired correctly (
onClick
vsonclick
typo?) - Use
debugger
insidehandleClick
to pause execution. - Check if any parent container is swallowing the event (like a
preventDefault
somewhere).
Building a Debugging Mindset
- Don't guess. Observe and investigate.
- Isolate issues. Comment out blocks to find culprits faster.
- Master DevTools. Especially the Console, Network, and Sources tabs.
- Read errors carefully. They usually tell you exactly what's wrong if you pay attention.
Tools to Amplify Your Debugging
- React DevTools — Visualize component hierarchy, props, and state.
- Redux DevTools — If using Redux, track actions and state changes easily.
- VSCode Debugger — Set breakpoints directly inside your code editor for seamless inspection.
Final Thoughts
Great developers aren't the ones who never face bugs. They're the ones who debug efficiently and learn faster from every problem.
Master these debugging fundamentals now, and you'll separate yourself from the average developers much faster!